Why React is important
In plain english: React might not last, there might be a better React tomorrow or next year but building user interfaces programmatically…
In plain english: React might not last, there might be a better React tomorrow or next year but building user interfaces programmatically with functions, that’s here to stay.
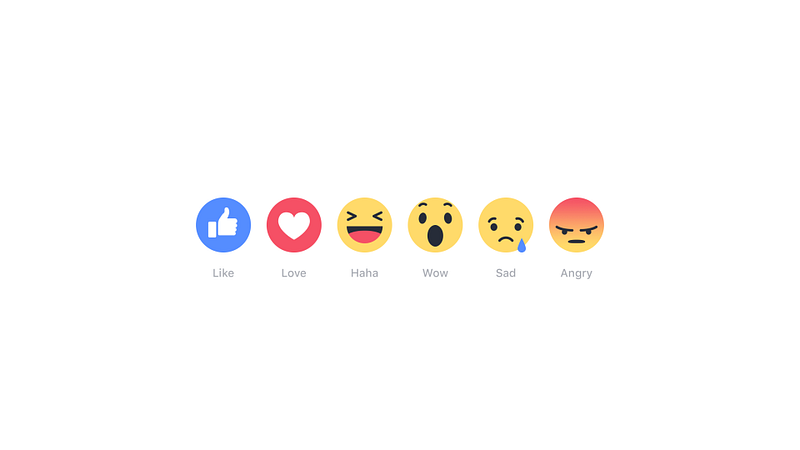
React is the new hotness, and truthfully I was hoping it would go away. I looked at the tutorial when it first came out and thought it was overly complex, didn’t fit with my view of the world (Rails) and felt like a company trying to lock me into a certain way of thinking.
I had had the same reaction to Angular when it burst on the scene too. While it felt a little more intuitive (Angular 1 of course), it too felt like Google trying to get me to be like Google. I was fine w/ Rails + jQuery.
I was wrong. React, is the future, or at least the underlying pattern that React implements is: functional programming of user interfaces.
What is functional programming? It’s a fancy way to say that if you have a certain set of inputs, and put them into a machine, you will always get the same outputs. If the inputs don’t change, the outputs won’t either.
f(x) = x + 3
If x = 7, then the answer is 10. It will always be 10 if x = 7.
Okay, now you understand functional programming, seriously it’s that simple. Let’s extend x + 3 into the world of user interfaces.
f(u) = header(x,y,z) + body(a,b,c) + footer(i,j,k)
Here is a function, f(u), that renders a user interface. The function is made up of other functions: header, body, and footer. Each of those functions take 3 parameters.
So what happens if your header and footer don’t change from request to request?
Remember above I said that a function x + 3 will always return 10 if x = 7 ? Why do you even need to compute the answer of 10, if you know that x = 7. If x doesn’t change, then you don’t need to run your function again.
At it’s core, this is how React works. It selectively calls functions that return outputs that represent user interface components. If the inputs don’t change, x + 3 = 10, therefore x = 7, then you don’t need to re-render that function. You know it will be 10, because the input is still 7.
I know this sounds brain dead simple for anyone who is using React in their day jobs, or has built something significant with it, but truthfully this is incredible. It is a better pattern than traditional Ajax. Not in a, “some people prefer this over that”, it’s just better.
And here is where many developers stop. That’s where I stopped. Yesterday, I grabbed coffee with one of my developer mentors, Anson. He’s building a new software workflow company and is using React on the front-end. He pushed me to think about the simple arguments above. I did, and a bit has flipped. Looking at the docs with my new simplified perspective, I “get it” even though I don’t “get” React.
Quick side note. Replace React here with Angular, Ember or whatever new front-end framework is really popular right now. I am picking React because Tilt uses it, Anson uses it and lots of other people do too. This article really isn’t about React, I just figured putting that in the title would get me an n+1 view, sorry. This is about the underlying functional pattern that React, and Angular implement. If you are worried about locking into Facebook’s view of the world, or Google’s or Tilde’s… who cares. If that’s your excuse it’s not a very good one. The libraries are popular because they are solving real problems, not because Zuck or Sergey told us to fork them.
Once you have digested the insanely simple argument above (the x = 7 and the answer is always 10 one), you should be able to see that traditional full page rendering + Ajax is dead. It’s gone, and it’s not coming back. Someone has built a better mousetrap. Knowing this, I can be honest with myself. What stopped me from jumping into React was fear. Not fear of not being good enough, or smart enough to learn it, but fear of the unknown.
I am really good at Rails + jQuery, and you probably are too. Or Django, or Node, or Java, or whatever code you are using to generate your views + jQuery. You are probably really good at caching, and expiring those caches and all that jazz… I am too. It’s not hard, well it’s kind of hard but you can figure it out.
I am not good at React, because the underlying pattern doesn’t feel natural to me. I am so used to making my apps a certain way. I love the bloated JS files I have. I love jQuery, I love weird ajax spaghetti code because it’s familiar and frankly, it’s easy. If someone describes an app to me, I can easily think through how to make it using Rails, jQuery, et al. I am trying to make a basic CRUD app right now in React it’s a f$&*king slog, but I have to do it.
Reacts is to functional programming of views as Rails was to MVC. It’s a great framework that implements a practical pattern. Fear not. Here we go.
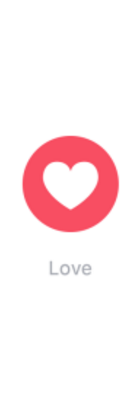